13
章节13
错误处理
在上一章中,您学习了如何使用服务器操作来修改数据。让我们看看如何使用 JavaScript 的 try/catch
语句和 Next.js API 来优雅地处理未捕获的异常。
本章内容...
以下是我们将涵盖的主题
如何使用特殊的 error.tsx
文件来捕获路由段中的错误,并向用户显示备用 UI。
如何使用 notFound
函数和 not-found
文件来处理 404 错误(针对不存在的资源)。
向服务器操作添加 try/catch
首先,让我们将 JavaScript 的 try/catch
语句添加到您的服务器操作中,以便您可以优雅地处理错误。
如果您知道如何操作,请花几分钟更新您的服务器操作,或者您可以复制下面的代码
请注意,redirect
是在 try/catch
块之外调用的。这是因为 redirect
通过抛出错误来工作,而该错误将被 catch
块捕获。为了避免这种情况,您可以在 try/catch
之后调用 redirect
。只有当 try
成功时,redirect
才会可达。
我们通过捕获数据库问题并从服务器操作返回有用的消息来优雅地处理这些错误。
如果您的操作中存在未捕获的异常会发生什么?我们可以通过手动抛出错误来模拟这种情况。例如,在 deleteInvoice
操作中,在函数顶部抛出一个错误
export async function deleteInvoice(id: string) {
throw new Error('Failed to Delete Invoice');
// Unreachable code block
await sql`DELETE FROM invoices WHERE id = ${id}`;
revalidatePath('/dashboard/invoices');
}
当您尝试删除发票时,您应该在 localhost 上看到错误。当进入生产环境时,您希望在发生意外情况时更优雅地向用户显示消息。
这就是 Next.js error.tsx
文件发挥作用的地方。请确保在测试后以及在继续下一节之前删除此手动添加的错误。
使用 error.tsx
处理所有错误
error.tsx
文件可用于为路由段定义 UI 边界。它充当意外错误的兜底,并允许您向用户显示备用 UI。
在您的 /dashboard/invoices
文件夹中,创建一个名为 error.tsx
的新文件并粘贴以下代码
'use client';
import { useEffect } from 'react';
export default function Error({
error,
reset,
}: {
error: Error & { digest?: string };
reset: () => void;
}) {
useEffect(() => {
// Optionally log the error to an error reporting service
console.error(error);
}, [error]);
return (
<main className="flex h-full flex-col items-center justify-center">
<h2 className="text-center">Something went wrong!</h2>
<button
className="mt-4 rounded-md bg-blue-500 px-4 py-2 text-sm text-white transition-colors hover:bg-blue-400"
onClick={
// Attempt to recover by trying to re-render the invoices route
() => reset()
}
>
Try again
</button>
</main>
);
}
关于上面的代码,您会注意到以下几点
- "use client" -
error.tsx
需要是一个客户端组件。 - 它接受两个属性
error
:此对象是 JavaScript 原生Error
对象的实例。reset
:这是一个重置错误边界的函数。执行后,该函数将尝试重新渲染路由段。
当您再次尝试删除发票时,您应该看到以下 UI
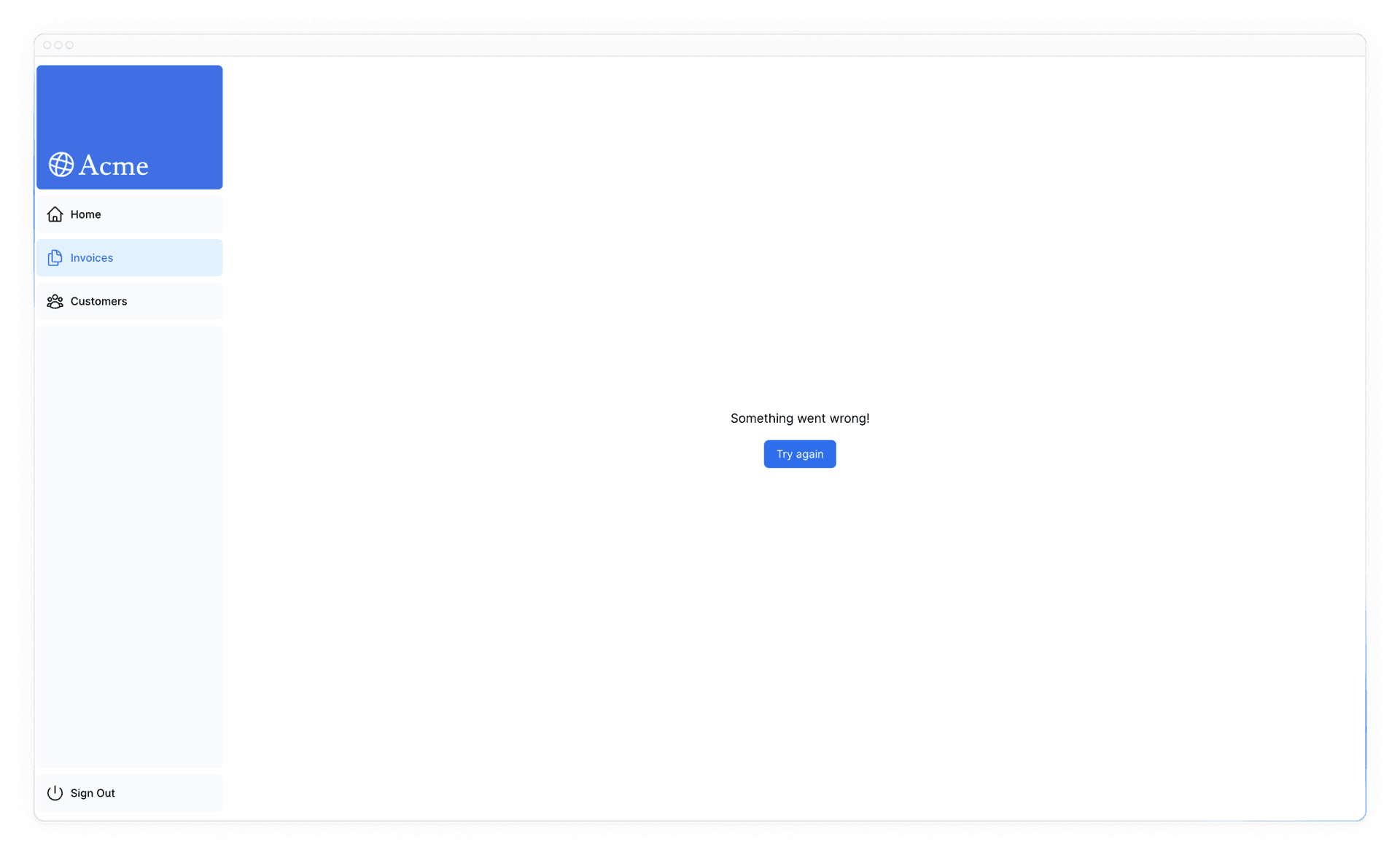
使用 notFound
函数处理 404 错误
另一种优雅处理错误的方法是使用 notFound
函数。虽然 error.tsx
对于捕获未捕获的异常很有用,但当您尝试获取不存在的资源时,可以使用 notFound
。
例如,访问 https://:3000/dashboard/invoices/2e94d1ed-d220-449f-9f11-f0bbceed9645/edit。
这是一个虚假的 UUID,在您的数据库中不存在。
您会立即看到 error.tsx
启动,因为这是定义了 error.tsx
的 /invoices
的子路由。
但是,如果您想更具体,您可以显示 404 错误,以告知用户他们尝试访问的资源未找到。
您可以通过进入 data.ts
中的 fetchInvoiceById
函数并控制台记录返回的 invoice
来确认资源未找到
export async function fetchInvoiceById(id: string) {
try {
// ...
console.log(invoice); // Invoice is an empty array []
return invoice[0];
} catch (error) {
console.error('Database Error:', error);
throw new Error('Failed to fetch invoice.');
}
}
现在您知道发票在您的数据库中不存在,让我们使用 notFound
来处理它。导航到 /dashboard/invoices/[id]/edit/page.tsx
,并从 'next/navigation'
导入 { notFound }
。
然后,您可以使用条件语句来调用 notFound
,如果发票不存在
import { fetchInvoiceById, fetchCustomers } from '@/app/lib/data';
import { notFound } from 'next/navigation';
export default async function Page(props: { params: Promise<{ id: string }> }) {
const params = await props.params;
const id = params.id;
const [invoice, customers] = await Promise.all([
fetchInvoiceById(id),
fetchCustomers(),
]);
if (!invoice) {
notFound();
}
// ...
}
然后,为了向用户显示错误 UI,在 /edit
文件夹内创建一个 not-found.tsx
文件。
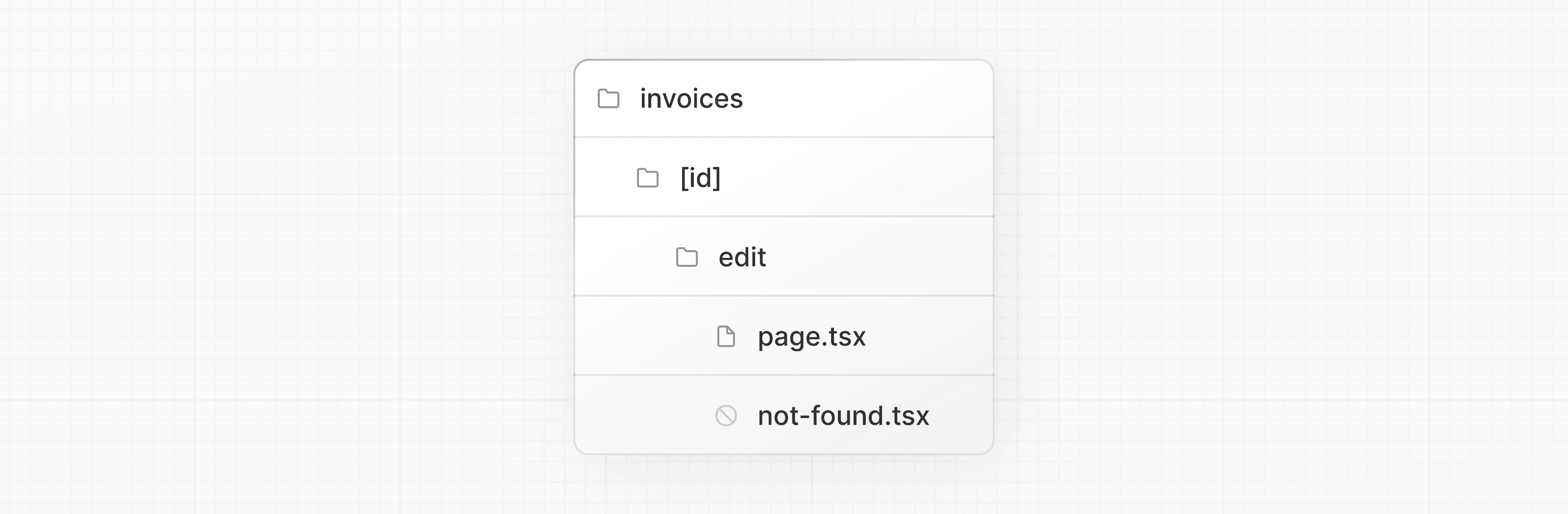
在 not-found.tsx
文件中,粘贴以下代码
import Link from 'next/link';
import { FaceFrownIcon } from '@heroicons/react/24/outline';
export default function NotFound() {
return (
<main className="flex h-full flex-col items-center justify-center gap-2">
<FaceFrownIcon className="w-10 text-gray-400" />
<h2 className="text-xl font-semibold">404 Not Found</h2>
<p>Could not find the requested invoice.</p>
<Link
href="/dashboard/invoices"
className="mt-4 rounded-md bg-blue-500 px-4 py-2 text-sm text-white transition-colors hover:bg-blue-400"
>
Go Back
</Link>
</main>
);
}
刷新路由,您现在应该看到以下 UI
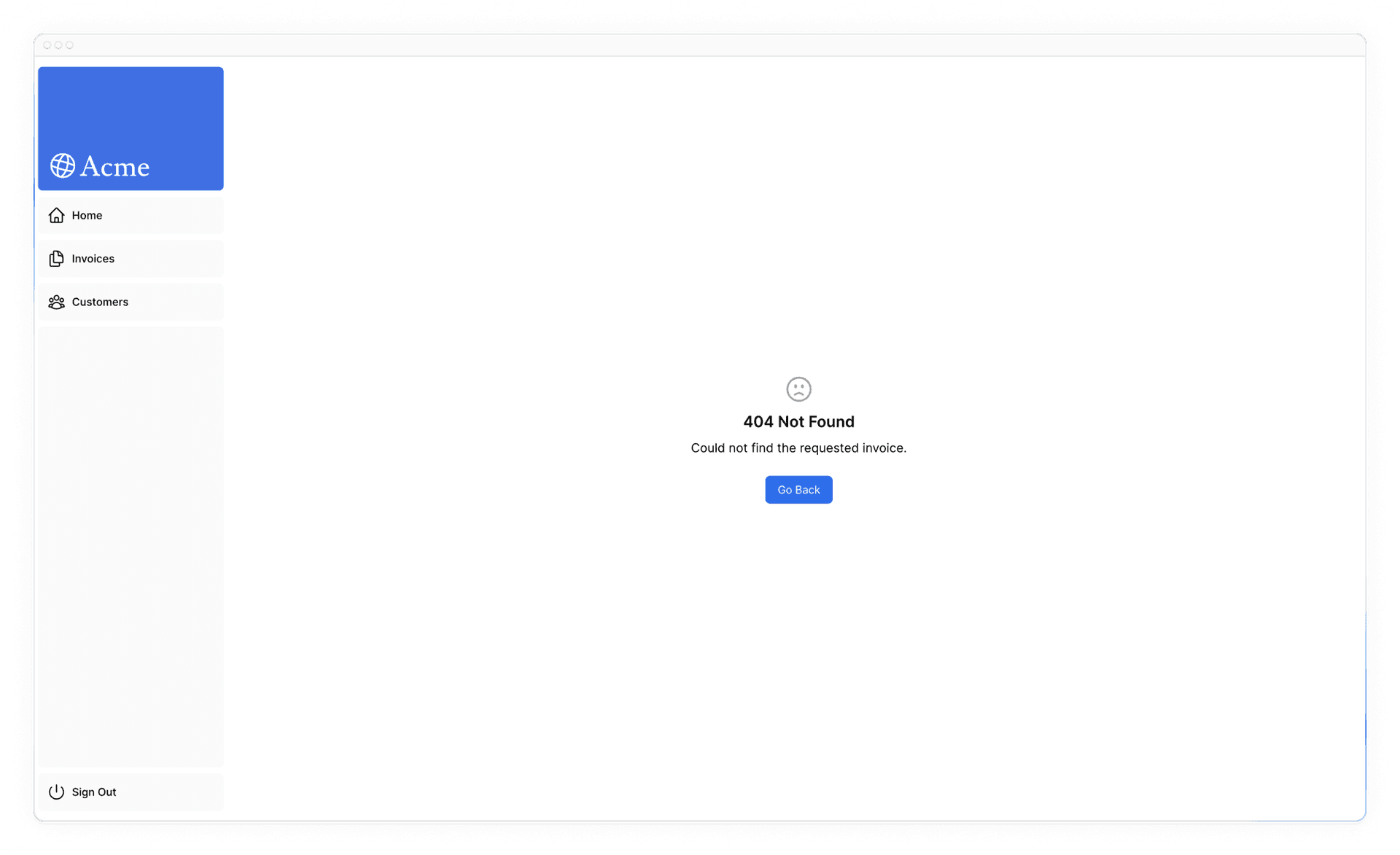
请记住,notFound
将优先于 error.tsx
,因此当您想要处理更具体的错误时,可以求助于它!
延伸阅读
要了解有关 Next.js 中错误处理的更多信息,请查看以下文档
这有帮助吗?