如何处理错误
错误可以分为两类:预期错误和未捕获的异常。本页将引导你了解如何在 Next.js 应用程序中处理这些错误。
处理预期错误
预期错误是指在应用程序正常运行期间可能发生的错误,例如来自服务器端表单验证或请求失败的错误。这些错误应显式处理并返回给客户端。
服务器操作
你可以使用 useActionState
Hook 来管理 服务器函数 的状态并处理预期错误。避免对预期错误使用 try
/catch
块。相反,你可以将预期错误建模为返回值,而不是抛出的异常。
app/actions.ts
'use server'
export async function createPost(prevState: any, formData: FormData) {
const title = formData.get('title')
const content = formData.get('content')
const res = await fetch('https://api.vercel.app/posts', {
method: 'POST',
body: { title, content },
})
const json = await res.json()
if (!res.ok) {
return { message: 'Failed to create post' }
}
}
然后,你可以将你的 action 传递给 useActionState
Hook,并使用返回的 state
来显示错误消息。
app/ui/form.tsx
'use client'
import { useActionState } from 'react'
import { createPost } from '@/app/actions'
const initialState = {
message: '',
}
export function Form() {
const [state, formAction, pending] = useActionState(createPost, initialState)
return (
<form action={formAction}>
<label htmlFor="title">Title</label>
<input type="text" id="title" name="title" required />
<label htmlFor="content">Content</label>
<textarea id="content" name="content" required />
{state?.message && <p aria-live="polite">{state.message}</p>}
<button disabled={pending}>Create Post</button>
</form>
)
}
服务器组件
当在服务器组件内部获取数据时,你可以使用响应来有条件地渲染错误消息或 redirect
。
app/page.tsx
export default async function Page() {
const res = await fetch(`https://...`)
const data = await res.json()
if (!res.ok) {
return 'There was an error.'
}
return '...'
}
未找到
你可以在路由段中调用 notFound
函数,并使用 not-found.js
文件来显示 404 UI。
app/blog/[slug]/page.tsx
import { getPostBySlug } from '@/lib/posts'
export default async function Page({ params }: { params: { slug: string } }) {
const post = getPostBySlug((await params).slug)
if (!post) {
notFound()
}
return <div>{post.title}</div>
}
app/blog/[slug]/not-found.tsx
export default function NotFound() {
return <div>404 - Page Not Found</div>
}
处理未捕获的异常
未捕获的异常是意外错误,表示在应用程序的正常流程中不应发生的错误或问题。这些应该通过抛出错误来处理,然后这些错误将被错误边界捕获。
嵌套的错误边界
Next.js 使用错误边界来处理未捕获的异常。错误边界捕获其子组件中的错误,并显示回退 UI,而不是崩溃的组件树。
通过在路由段内添加 error.js
文件并导出一个 React 组件来创建错误边界
app/dashboard/error.tsx
'use client' // Error boundaries must be Client Components
import { useEffect } from 'react'
export default function Error({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
useEffect(() => {
// Log the error to an error reporting service
console.error(error)
}, [error])
return (
<div>
<h2>Something went wrong!</h2>
<button
onClick={
// Attempt to recover by trying to re-render the segment
() => reset()
}
>
Try again
</button>
</div>
)
}
错误将冒泡到最近的父错误边界。这允许通过在路由层级结构中的不同级别放置 error.tsx
文件来实现细粒度的错误处理。
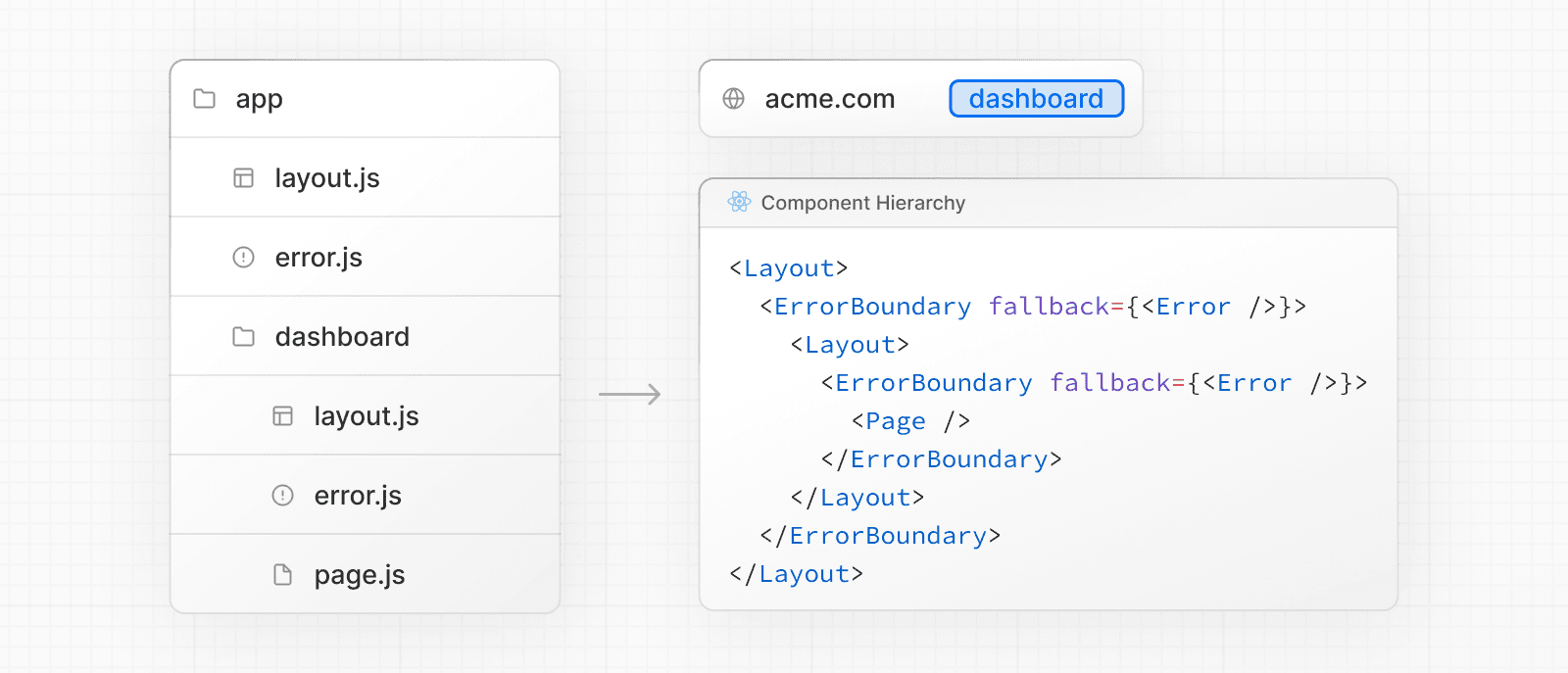
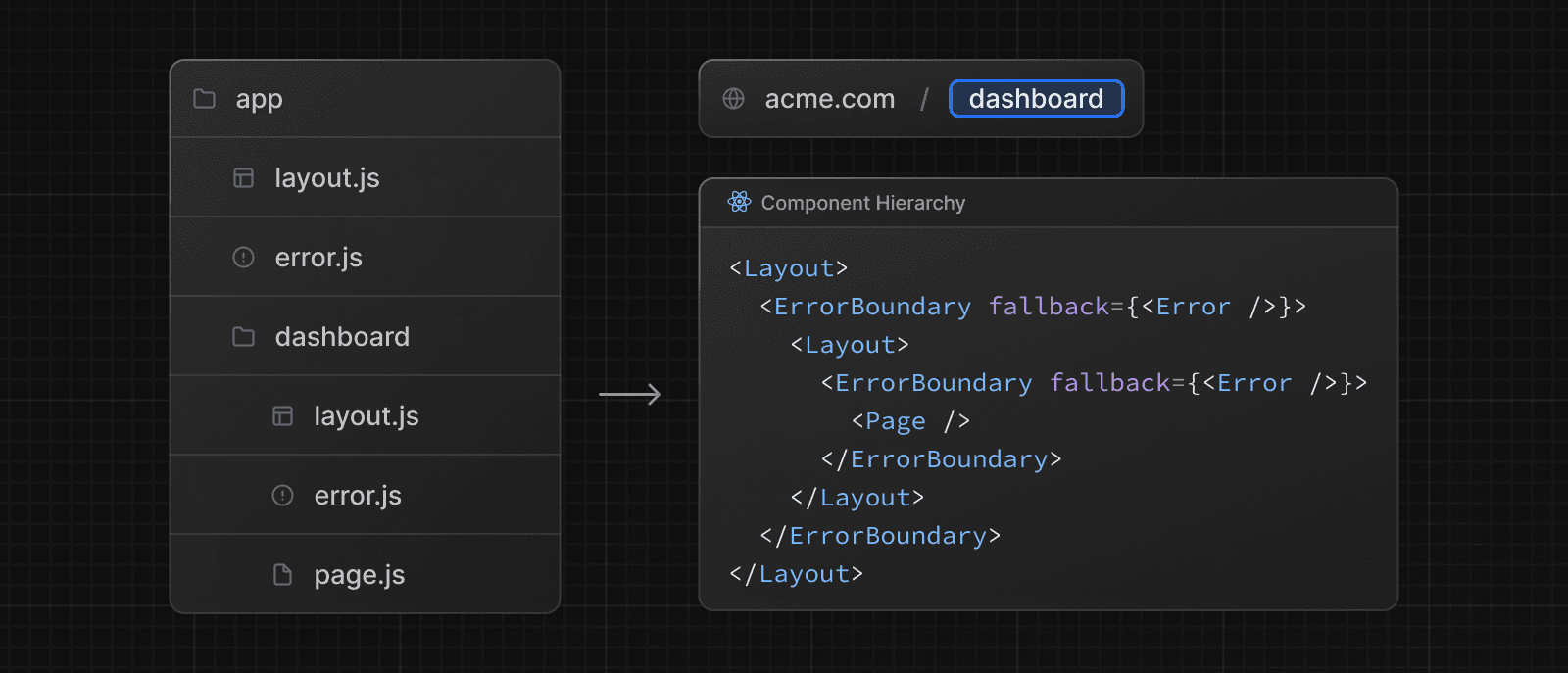
全局错误
虽然不太常见,但即使在使用国际化时,你也可以使用位于根 app 目录中的 global-error.js
文件在根布局中处理错误。全局错误 UI 必须定义自己的 <html>
和 <body>
标签,因为它在激活时会替换根布局或模板。
app/global-error.tsx
'use client' // Error boundaries must be Client Components
export default function GlobalError({
error,
reset,
}: {
error: Error & { digest?: string }
reset: () => void
}) {
return (
// global-error must include html and body tags
<html>
<body>
<h2>Something went wrong!</h2>
<button onClick={() => reset()}>Try again</button>
</body>
</html>
)
}
API 参考
通过阅读 API 参考,了解更多关于本页中提到的功能。
这篇文章对您有帮助吗?